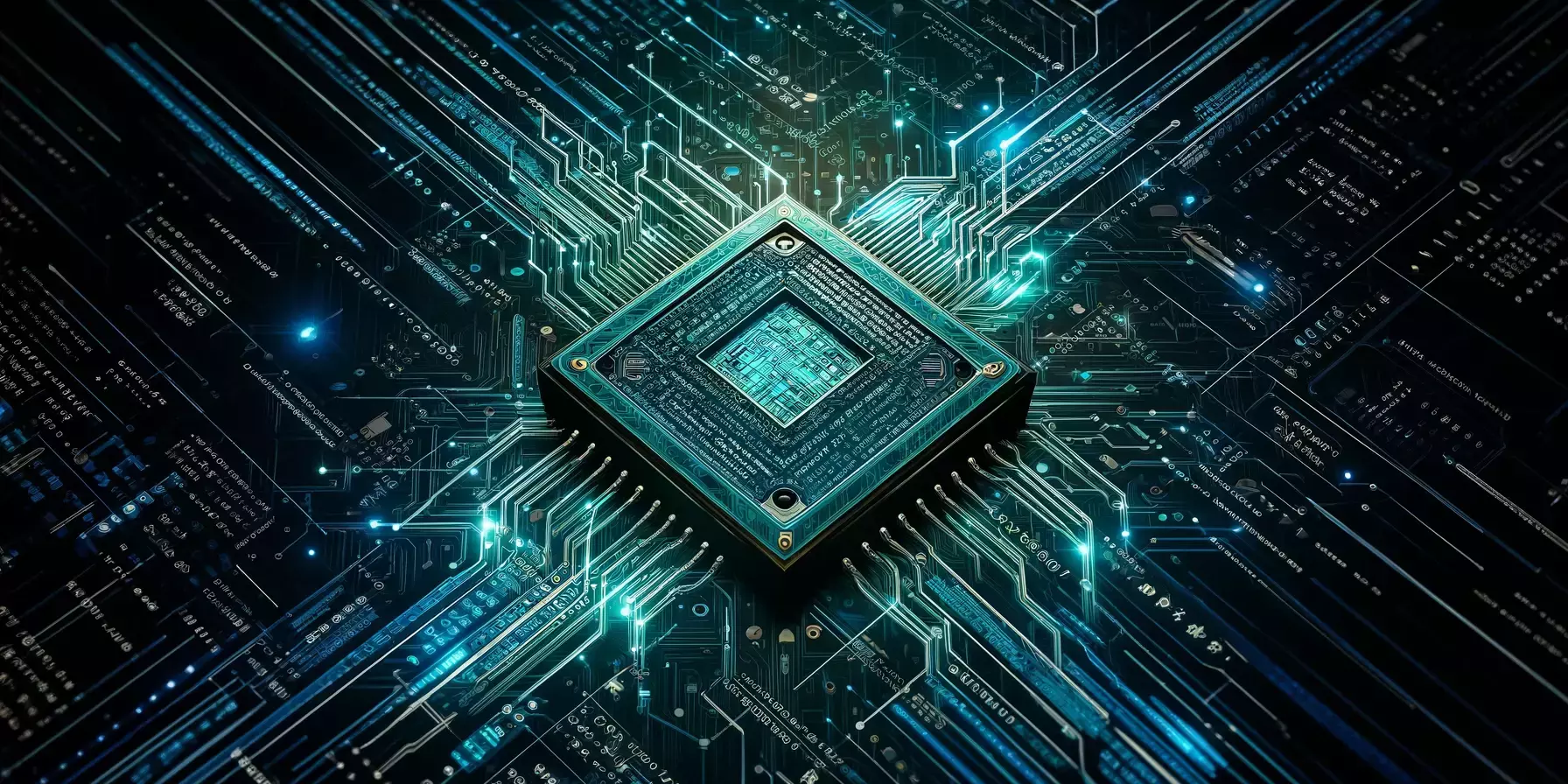
Generative AI is an exciting field that combines creativity with the power of artificial intelligence. Python, with its rich ecosystem of libraries and frameworks, is an excellent language for implementing Generative AI projects. This blog explores some intriguing Generative AI projects in Python, providing you with practical examples to get started on your AI journey.
Key Generative AI Python Projects
1. Text Generation with GPT‑3: GPT‑3, developed by OpenAI, is a powerful language model capable of generating human-like text. You can use the openai Python library to access GPT‑3 and create applications like chatbots, story generators, or even code completions. Here’s a simple example:
python
import openai
openai.api_key = ‘your-api-key’
response = openai.Completion.create(
engine=“text-davinci-003”,
prompt=“Once upon a time”,
max_tokens=50
)
print(response.choices[0].text.strip())
2. Image Generation with GANs: Generative Adversarial Networks (GANs) are a popular method for generating images. The tensorflow and keras libraries provide excellent tools for implementing GANs. Here’s a basic example of setting up a GAN for image generation:
python
import tensorflow as tf
from tensorflow.keras import layers
# Define the generator model
def build_generator():
model = tf.keras.Sequential([
layers.Dense(256, input_dim=100, activation=‘relu’),
layers.Reshape((16, 16, 1)),
layers.Conv2DTranspose(128, (4,4), strides=(2,2), padding=‘same’, activation=‘relu’),
layers.Conv2DTranspose(64, (4,4), strides=(2,2), padding=‘same’, activation=‘relu’),
layers.Conv2D(1, (7,7), activation=‘sigmoid’, padding=‘same’)
])
return model
generator = build_generator()
generator.summary()
3. Music Generation with RNNs: Recurrent Neural Networks (RNNs) are effective for generating sequential data like music. Libraries like music21 and tensorflow can be used to create models that compose music. Here’s a basic example of setting up an RNN for music generation:
python
import music21
import tensorflow as tf
from tensorflow.keras import layers
# Define the RNN model
def build_rnn():
model = tf.keras.Sequential([
layers.LSTM(128, input_shape=(100, 1), return_sequences=True),
layers.LSTM(128),
layers.Dense(256, activation=‘relu’),
layers.Dense(128, activation=‘softmax’)
])
return model
rnn_model = build_rnn()
rnn_model.summary()
4. Style Transfer with Neural Networks: Neural style transfer involves using deep learning to apply the artistic style of one image to another. The tensorflow and keras libraries make this process straightforward. Here’s an example of setting up a style transfer model:
python
import tensorflow as tf
# Load pre-trained VGG19 model + higher level layers
vgg = tf.keras.applications.VGG19(include_top=False, weights=‘imagenet’)
# Create the model for style transfer
def build_style_transfer_model():
model = tf.keras.models.Model([vgg.input], [vgg.get_layer(‘block5_conv2’).output])
return model
style_transfer_model = build_style_transfer_model()
style_transfer_model.summary()
Best Practices for Implementing Generative AI in Python
1. Quality of Data: The success of Generative AI models heavily relies on the quality of the training data. Ensure that your datasets are diverse, representative, and pre-processed correctly to achieve the best results.
2. Model Training and Fine-Tuning: Training generative models requires significant computational resources and time. Fine-tuning pre-trained models can save time and improve performance, especially when working with limited data.
3. Ethical Considerations: Generative AI has the potential to produce misleading or biased content. Implement safeguards to ensure ethical AI use, such as monitoring outputs and applying filters to prevent harmful content generation.
Conclusion
Generative AI in Python opens up a world of creativity and innovation. Whether you’re generating text, images, music, or artistic styles, Python’s versatile libraries provide the tools you need to bring your AI projects to life. By following best practices and experimenting with different models, you can harness the power of Generative AI to create remarkable applications.